CloakShare - Unveiling the Fusion of Rust, Python, and React for Image Steganography
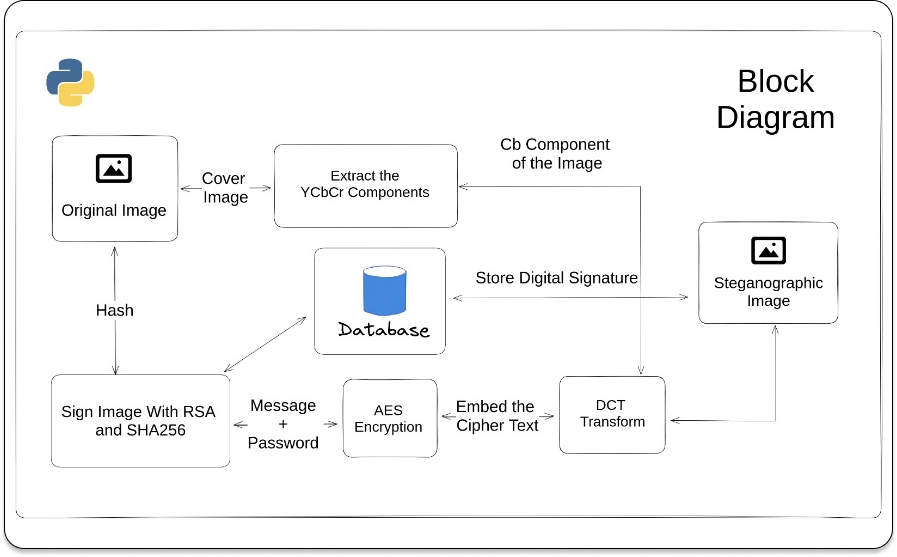
CloakShare - End to End Encryption Native Application built using Tauri
Introduction
This project for the course Cryptography and Network Security, created an end to end secure file transfer application secured by RSA and AES algorithms.
The app performs Image Steganography using the DCT method. Comparative analysis with other methods and easy to use desktop application with focus on UI/UX.
Frameworks Used
Rust and Python Backend
rust
//src-tauri/src/main.rslet output = std::process::Command::new("python").arg("D:\\Steganography-Encrpytion-App-main\\backend\\src\\main.py").arg("-e").arg(path).arg(output_path).arg(message).arg(pass).output().expect("failed to execute process");
rust
//src-tauri/src/main.rslet output = std::process::Command::new("python").arg("D:\\Steganography-Encrpytion-App-main\\backend\\src\\main.py").arg("-e").arg(path).arg(output_path).arg(message).arg(pass).output().expect("failed to execute process");
The rust backend calls the opencv
python script and passes the arguments to it
by using the std::process
library and gets the encrypted image back.
Rust (Tauri) and React (Vite)
rust
//src-tauri/src/main.rs#[tauri::command]fn steganography(path: &str, message: &str, pass: &str, app: tauri::AppHandle) -> Result<(), String> {...let mut file = File::open(output_path_clone).unwrap();let mut contents = Vec::new();file.read_to_end(&mut contents).unwrap();let contents = base64::encode(contents);let contents = format!("data:image/png;base64,{}", contents);app.emit_all("stegImage", Payload {message: contents,}).unwrap();Ok(())}
rust
//src-tauri/src/main.rs#[tauri::command]fn steganography(path: &str, message: &str, pass: &str, app: tauri::AppHandle) -> Result<(), String> {...let mut file = File::open(output_path_clone).unwrap();let mut contents = Vec::new();file.read_to_end(&mut contents).unwrap();let contents = base64::encode(contents);let contents = format!("data:image/png;base64,{}", contents);app.emit_all("stegImage", Payload {message: contents,}).unwrap();Ok(())}
Here we are compressing the image in base64 format and sending it to the react
using the tauri::AppHandle
and app.emit_all
function.
javascript
//src/App.jsximport { listen } from "@tauri-apps/api/event";...function App(){...useEffect(() => {listen("stegImage",(event) => {console.log(event.payload);setStegImage(event.payload.message as string);}).then((unlisten) => {return () => {unlisten();};});}, []);...return(...<buttononClick={() => {invoke("steganography", {path: filePath,message: message,pass: password,}).then((res) => {console.log(res);}).catch((err) => {console.log(err);});}} >...</button>...)}
javascript
//src/App.jsximport { listen } from "@tauri-apps/api/event";...function App(){...useEffect(() => {listen("stegImage",(event) => {console.log(event.payload);setStegImage(event.payload.message as string);}).then((unlisten) => {return () => {unlisten();};});}, []);...return(...<buttononClick={() => {invoke("steganography", {path: filePath,message: message,pass: password,}).then((res) => {console.log(res);}).catch((err) => {console.log(err);});}} >...</button>...)}
Here we are listening to the stegImage
event that is emitted by tauri and sets the required image in the react frontend.